Static Variable
Static Variable is achieved by using Static Keyword before it. Static Variable is also called as class variable. Static variable is associated with a class and not with object.Code to create Static Variable
public class CountNum { private int Number; public static int count=0; public CountNum () { count++; Number=count; } }
In this example, static keyword is used before count. So here count is static.Now for every object created, variable Number will assigned by a unique value, starting from 1 and counting upwards. This means variable count is shared among all instances of class CountNum.
A static variable is similar way to global variable. Static variable is a variable that is accessible from any instance of the class. If static variable is not marked as private then you can also access it from outside the class.
Static Method
Static method is achieved by using static keyword before method name while defining. Static method is also called as class method.
Code to create Static method in Java
public class CountNum2 { private int Number; public static int count=0; public static int TotalCount () { return count; } public CountNum2 () { count++; Number=count; } }If method is static than we have to access static method using the class name rather than an object reference. As Example:-
public class ExampleCount { public static void main(String[] args) { System.out.println("Number of Count is " +CountNu2.TotalCount() ); } }Output is:- Number of Count is 0 You can invoke a static method without any instance of the class. If you use static method then you should be aware about following facts: 1) You can not override static method but you can hide it. 2) The main() method is static method because the JVM does not create an instance of the class when executing the main method.
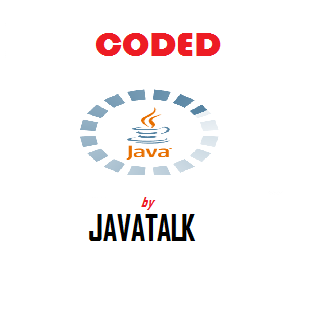
0 comments:
Post a Comment